APIs are the backbone of modern applications, acting as the bridge between client-side applications and backend servers.
When it comes to API design, two dominant approaches have emerged : REST and GraphQL. Both methods provide powerful ways to request and manage data, but they follow fundamentally different design philosophies.
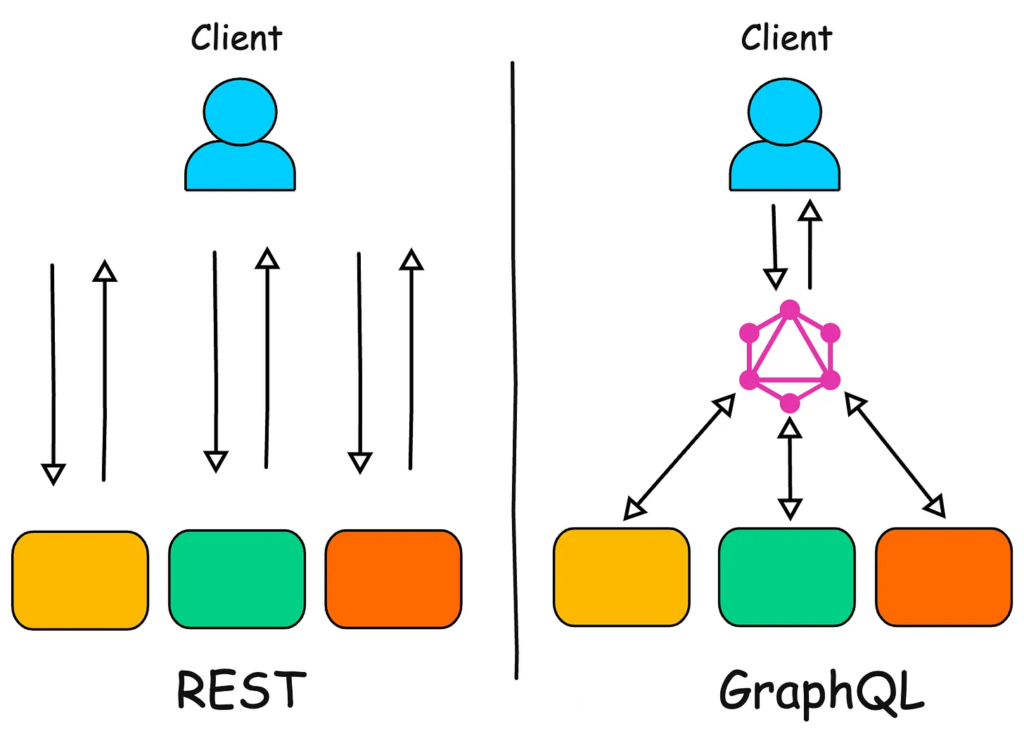
REST is a well established architectural style that relies on fixed endpoints and HTTP methods. Its simplicity and predictability have made it the industry standard for years.
On the other hand, GraphQL, developed by Facebook in 2015, takes a more dynamic and efficient approach. It allows clients to request exactly the data they need in a single query, reducing both over-fetching and under-fetching.
In this article, we’ll explore the differences between REST and GraphQL, highlight their pros and cons, and help you decide which one fits your project best.
What is REST?
REST (Representational State Transfer) is an architectural style introduced in the early 2000s for designing networked applications. It’s not a protocol but a set of guidelines that rely on the existing HTTP framework for communication between clients and servers.
How REST Works
REST revolves around resources, where each resource (like a user, order, or product) is identified by a unique URL. Clients interact with these resources using standard HTTP methods:
- GET : Retrieve a resource (e.g.,
GET /api/users/123
to fetch user data). - POST : Create a new resource (e.g.,
POST /api/users
to add a user). - PUT/PATCH : Update an existing resource (e.g.,
PUT /api/users/123
to modify user details). - DELETE : Remove a resource (e.g.,
DELETE /api/users/123
to delete a user).
Example
If you need to retrieve information about a user with ID 123
, you would make this request:
GET /api/users/123
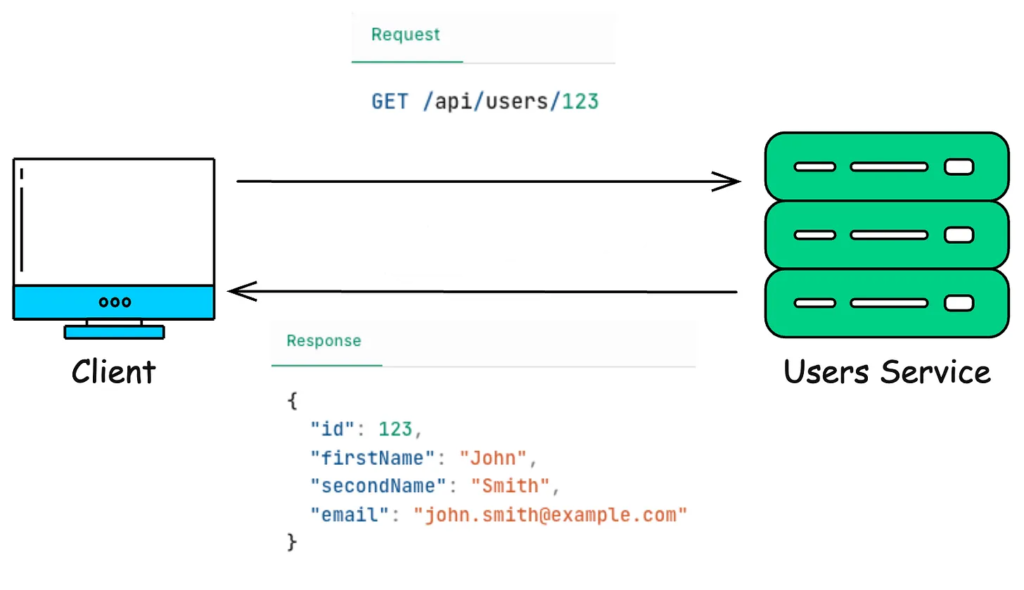
The server would respond with a JSON object containing the user’s data.
REST Status Codes
REST APIs typically return standard HTTP status codes to indicate the outcome of a request:
- 200 OK – Success
- 201 Created – Resource successfully created
- 400 Bad Request – Invalid client request
- 404 Not Found – Resource doesn’t exist
- 500 Internal Server Error – Server issue
Advantages of REST
Simple and Intuitive : REST’s resource-based model aligns well with most business use cases, making it easy to understand and implement.
Stateless : Each request is independent, improving scalability across distributed systems.
Built-in Caching : REST APIs can use HTTP caching to enhance performance.
Scalable : REST works well with load balancers and CDNs for high traffic scenarios.
Mature Ecosystem : REST has been widely used for over two decades, leading to strong tooling and community support.
Limitations of REST
Over-fetching : REST often returns more data than needed. For example, if a client only needs a user’s name and email, the API may also return the address, phone number, and other irrelevant fields.
Under-fetching : When the client needs related data (e.g., user details and user’s posts), multiple requests may be required
Versioning Challenges : Changes in the API often require versioning (e.g., /v1/users
vs /v2/users
), adding maintenance complexity.
Rigid Response Structure : The server defines how data is structured, limiting client-side flexibility.
What is GraphQL?
As applications grew more complex, REST’s limitations became more evident. To address these challenges, Facebook introduced GraphQL in 2015 as a more flexible and efficient data-fetching solution.
How GraphQL Works
Unlike REST, which relies on fixed endpoints, GraphQL exposes a single endpoint (/graphql
) where clients can define the data they need. This prevents over-fetching and under-fetching by allowing clients to request only the necessary fields.
Example
A GraphQL query to fetch a user’s name, email, and posts looks like this:
query {
user(id: 123) {
name
posts {
title
content
}
}
}
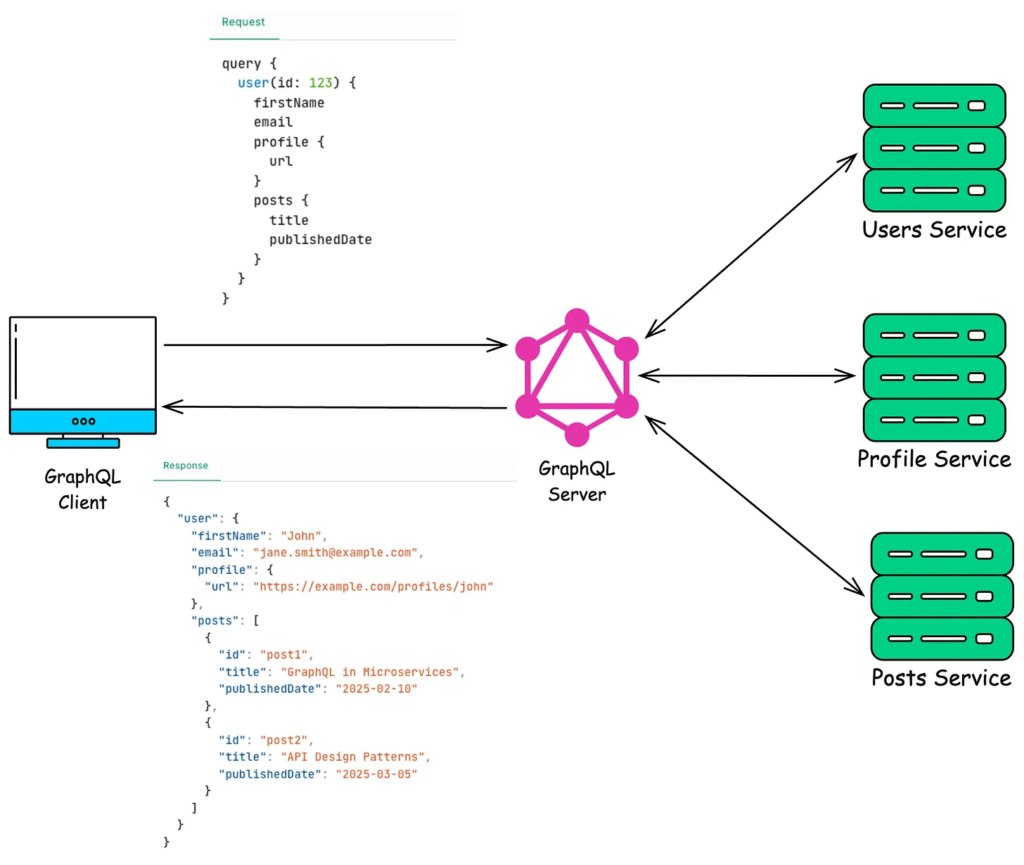
GraphQL allows the client to request data from multiple sources and aggregate it into a single response.
GraphQL Schema
GraphQL uses a strict schema to define data structures and relationships:
User {
id: ID!
firstName: String!
lastName: String!
email: String!
posts: [Post!]
}
type Post {
id: ID!
title: String!
content: String!
}
type Query {
user(id: ID!): User
posts: [Post!]!
}
Core Features of GraphQL
- Queries : Request specific data fields.
- Mutations : Create, update, or delete data.
- Subscriptions : Enable real-time updates.
Example : Creating a New Post
mutation {
createPost(title: "GraphQL vs REST", content: "GraphQL solves REST’s limitations...") {
id
title
content
}
}
Example : Real-Time Updates
subscription {
newPost {
title
content
author {
name
}
}
}
Advantages of GraphQL
Precise Data Fetching : Clients get exactly what they request—nothing more, nothing less.
Single Request for Multiple Resources : Multiple data sources can be queried simultaneously.
Strong Typing : Schema-based structure improves discoverability and documentation.
Real-Time Data : Subscriptions enable automatic data updates.
Flexible API Evolution : New fields can be added without breaking existing queries.
Limitations of GraphQL
❌ Complex Setup : GraphQL requires a specialized server, schema, and resolvers.
❌ Caching Challenges : GraphQL queries are usually POST requests, making HTTP caching harder.
❌ Increased Server Load : Clients can request large amounts of data, potentially overloading the server.
❌ Security Risks : Deeply nested queries can lead to performance bottlenecks and DoS attacks.
REST vs GraphQL – Key Differences
Feature | REST | GraphQL |
---|---|---|
Data Structure | Resource-based | Schema-based |
Data Fetching | Fixed responses | Client-defined responses |
Over-fetching/Under-fetching | Common issue | Avoided |
Real-time Updates | Difficult | Built-in with subscriptions |
API Versioning | Often required | Not needed |
Caching | Easy with HTTP | More complex |
Setup Complexity | Simple | Higher |
When to Use REST vs GraphQL
Use REST if:
- Your API is simple with predictable data needs.
- HTTP caching is important.
- You need a standardized and well-established approach.
Use GraphQL if:
- You need precise and efficient data fetching.
- Your app has complex, nested data structures.
- Real-time updates are required.
- You want to avoid versioning issues.
Can You Use Both REST and GraphQL?
Absolutely! Many companies combine REST and GraphQL to get the best of both worlds:
- GraphQL – For flexible, client-facing data fetching.
- REST – For simple, predictable internal services.
👉 Thank you for reading!
If you found it valuable, hit a like ❤️ and consider subscribing for more such content every week.